Today we are going to talk about HTML and CSS, showing some fundamentals that they offer and how we use these markups in our pages and, for that, we are going to build a simple page that generates sharing links to various web tools.
As always, the used and commented examples are in our GitHub repository and also on our CodePen that we use to show the real-time versions here.
Hello, if this is your first time here, our content is geared towards INNOVATION (and for those who like technology/programming and are just starting out or are from other fields) and, in particular, this series is aimed at PROGRAMMING FOR BEGINNERS.
I will also post a video on this topic on my YouTube channel, which is where I share some thoughts, tips and projects I am currently working on with other tech-fanatics, whether you are a developer or enthusiast. Please also consider subscribing to my channel to not miss future videos and to join our live "coding sessions" and a nice, relaxing tech-related chat -- I promise to keep things interesting. The content is in Portuguese, but you can watch my videos with subtitles (maybe I will switch to English in the future, if this makes sense).
Once I publish the video, I will update this post to reflect the content.
All set? Then tag along!
What is HTML and CSS?
Even if you're new to the web development world, you've probably heard of HTML (Hypertext Markup Language). What about CSS (Cascading Style Sheets), heard of it?
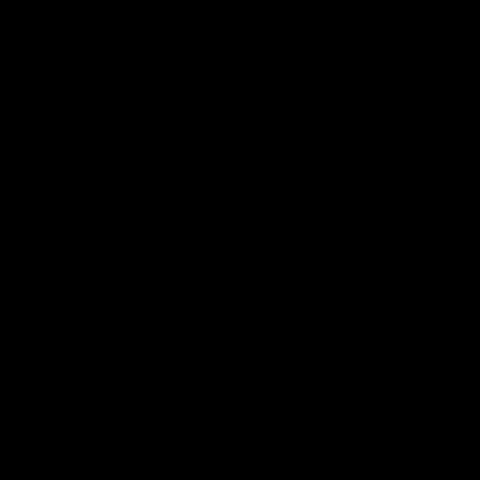
There's a ton of discussions if knowing HTML is knowing programming (spoiler, it's not!) but the fact is that everyone dedicated to web development needs to know how HTML and CSS works to build applications that run in the browser. In a way it is possible to say that HTML and CSS are the "backbone" of web development.
For beginners, learning the basics of HTML and CSS is a must and will certainly be useful in any web application you need to develop.
How does HTML work?
When you access a website through an Internet browser (Chrome, Firefox, Internet Explorer), that browser will look for a file that contains code and will be interpreted before being displayed to you.
This file that contains code can have several possible extensions, depending on the technology used on the site (such as asp, php) but, in the end, even if there are other possible technologies, the browser will receive an HTML code.
In the simplest case and without other technologies involved, you can have a page with a .htm or .html extension that contains the HTML code and your browser will render it.
HTML Tags
Browsers are not very "picky" to render HTML code, as it is a markup syntax and not a programming language itself.
For example, if you create files on your computer called "Test_1.html", "Test_2.html" and "Test_3.html", and place the following contents one at a time, they will all open in the browser in the same way:
Test_1.html
See the Pen Test_1.html by Octavio Ietsugu | Dev.Lawyer (@devlawyer) on CodePen.
Test_2.html
See the Pen Test_2.html by Octavio Ietsugu | Dev.Lawyer (@devlawyer) on CodePen.
Test_3.html
See the Pen Teste_3.html by Octavio Ietsugu | Dev.Lawyer (@devlawyer) on CodePen.
As you can see, the content of these three files is different, but the result will be the same.
See these words inside the greater of and lesser of signs? These are HTML tags and they indicate where some item of your code starts and where it ends (those that end with "/"). For example, when we type <p> we want to indicate that there is a paragraph there, and that it goes as far as the </p> appears.
And this is more or less the principle for the other HTML tags.
Some tags like "<img>" (to insert an image) don't have the closing tag (it doesn't have the "</img>") and in these cases you can "close" the tag itself. putting the "/" at the end of the tag, like this:
Basic HTML Tags
To assemble an HTML document you can use the most common HTML tags that will possibly meet most of your needs -- see the example below that contains these most common tags:
See the Pen example_basichtmlpage.html by Octavio Ietsugu | Dev.Lawyer (@devlawyer) on CodePen.
In this simple HTML document we have the following (these comments are also in the document inside our repo):
<!DOCTYPE html> Tells the browser we are dealing with an HTML file
<html> Indicates that the HTML document starts here
<head> Brings information that is needed by the document but that
do not represent elements on the rendered page. We can
include here, for example, scripts, CSS styles, information
about the title, links and meta-tags.
<title> It is the title of the page and that appears in the browser tab
<body> Here begins the "body" of the document, and this is where
all the elements that should appear on the rendered page should be.
<div> One of the most used elements mainly for organization of elements
on the screen, the div is like a "division" of the page. By placing
a <div> your page will have that element that represents a division
in the document that has some content that should be displayed.
<h1> It is a header in the document (header), with h1 being the largest
and h6 the smallest.
<em> Makes the text highlighted (usually italics and could be <i> too,
but <em> is better for accessibility as it can be interpreted
in reading softwares)
<strong>Make the text bold (could be <b> too, but strong is better
for accessibility)
<br /> Skip a line
<p> One paragraph
<span> It is also a division, but this one occurs on the line itself. It
segments the text.
<a> Represents a link
<img /> Represents an image
<input /> Input data field (form)
<ul> Unordered list (bullet points)
<ol> Ordered (numbered) list
<li> List Item
<table> table
<tr> Table row
<td> Table column
<!-- -->Comment tags: everything inside this tags will not be rendered
Other HTML Tags: "Advanced HTML"
When talking about HTML, it is common for developers to end up simplifying everything and throwing only the most common elements. For example, on any page you come across you will likely find a series of <div> elements as they are used to create divisions on the page.
This is not wrong in itself and stems from the way HTML worked before HTML5, but there are advantages in using specific tags for different parts of the document, especially when it comes to user accessibility (visually impaired users, for example) and even on the reading of your document by search engines.
But did you know that HTML, and especially HTML5, has many interesting functions "up" your page? We're going to list some of them here in an "Advanced HTML" mode, if I may say so 🤣
HTML5 Tags
With HTML5, the so-called Semantic Tags were introduced, which aim to describe some code blocks that have specific functions on the page and that are quite common. Before HTML5 everything was done in <div> but it is good practice to use semantic tags when possible.
When placing these tags without any other styling, you will only see lines one below the other. To make the correct use of these tags, you must complement them with the rest of the page's visuals for a correct layout (read CSS, which we will cover later).
See the Pen example_html5page.html by Octavio Ietsugu | Dev.Lawyer (@devlawyer) on CodePen.
And in this document with the semantic tags we have the following:
<header> Header of the page, where you can place a banner
for example
<nav> Navigation: usually a navigation menu
<aside> Side menu
<main> Location of the main content of the page
<section> If there are no more specific semantic tags, you can
can use a <section> to indicate a section on the page.
<footer> Footer of the page
More interesting HTML Tags and Attributes
Today we have much more possibilities with HTML than you could have imagined years ago. And this is very positive because it eliminates the need to use Javascript, for example, in a lot of cases.
Let's see some interesting HTML tags and attributes:
See the Pen example_unusualhtml.html by Octavio Ietsugu | Dev.Lawyer (@devlawyer) on CodePen.
Need more? I've found a very interesting site that has a lot of references and HTML examples that you can use:
https://htmlcheatsheet.com/The HTML + CSS combo is what will bring your web page closer to the final result.
When you assemble the HTML, you are actually putting together the structure and content of your page. There you define the elements that make up the page, text and images that it should load. But still, this is "raw" text, like a skeleton. To make your page look more refined, you will need to use CSS.
CSS allows you to style each of the HTML elements on your page, giving it visual properties that HTML alone cannot provide.
To use CSS in your HTML you have three options:
- Inline CSS
- Internal CSS
- External CSS
Inline CSS
In Inline CSS you use the styles you want inside the HTML element with a style attribute, as follows:
<p style="font-weight:bold">Sample text</p>
Internal CSS
In Internal CSS we can add a <style> tag inside the <head> tag in HTML, defining the desired styles there.
<style>
p{
font-weight: bold;
}
</style>
<p>Sample text</p>
External CSS
Finally, we can include an External CSS file that contains the desired styles by placing a <link> tag inside the <head> tag.
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<link rel='stylesheet' type='text/css' href='main.css'>
</head>
<body>
<p>Sample text</p>
</body>
</html>
main.css
Regardless of the way you decide to use CSS on your page, just know that basically you need to indicate the element, element type or class of an element that will be visually changed and, after that, indicate which properties will be changed. Basically:
See the Pen example_basiccss.html by Octavio Ietsugu | Dev.Lawyer (@devlawyer) on CodePen.
Working with CSS allows you to do a LOT of things, taking your HTML to the next level. In some upcoming content we'll talk a little more about CSS and I'll show you how it's possible to do very extreme things with CSS that you can't even imagine!
CREATING A SIMPLE HTML AND CSS PROJECT
And now that we've finally covered the basics of how HTML and CSS work, let's get down to business and put together a simple project that uses mostly HTML and CSS, bringing something useful, however small it may be.
For our project, we are going to create a website that receives a text and prepares the HTML code so that the user can create a link to share that text on different social media: WhatsApp, Facebook, Twitter, etc. The program itself is very simple, but it is a good opportunity to show the application of HTML and CSS concepts in practice.
What does this project do?
In fact, if you already have this list, your problem is solved, but I took advantage of the idea to build a very simple program showing how we can use HTML to build a simple software structure, CSS to give it a better aesthetic layer and finally a little bit of Javascript to give some functionality. As always, the code is all commented with some points that I thought were relevant so that you can follow what was done.

Analyze the index.html file to find all the elements that make up our page, such as buttons, texts and input fields.
In the main.css file you will find the styles used in the text and icons present on the screen.
Finally, in the main.js file you will find some functions that make our program useful, allowing the program to be more interactive with what is provided on the screen.
I recommend that you read the comments I left in the files and interpret the code as well. Analyzing other people's code is a great way to learn more about programming. Suggestions are always welcome. 😀
/* End of post, see you next time! */
Thank you for providing this article Web Development Toronto. Through it, I gained some useful knowledge. Thank you for writing such a great post.
ReplyDelete